how to draw open front a 3d box
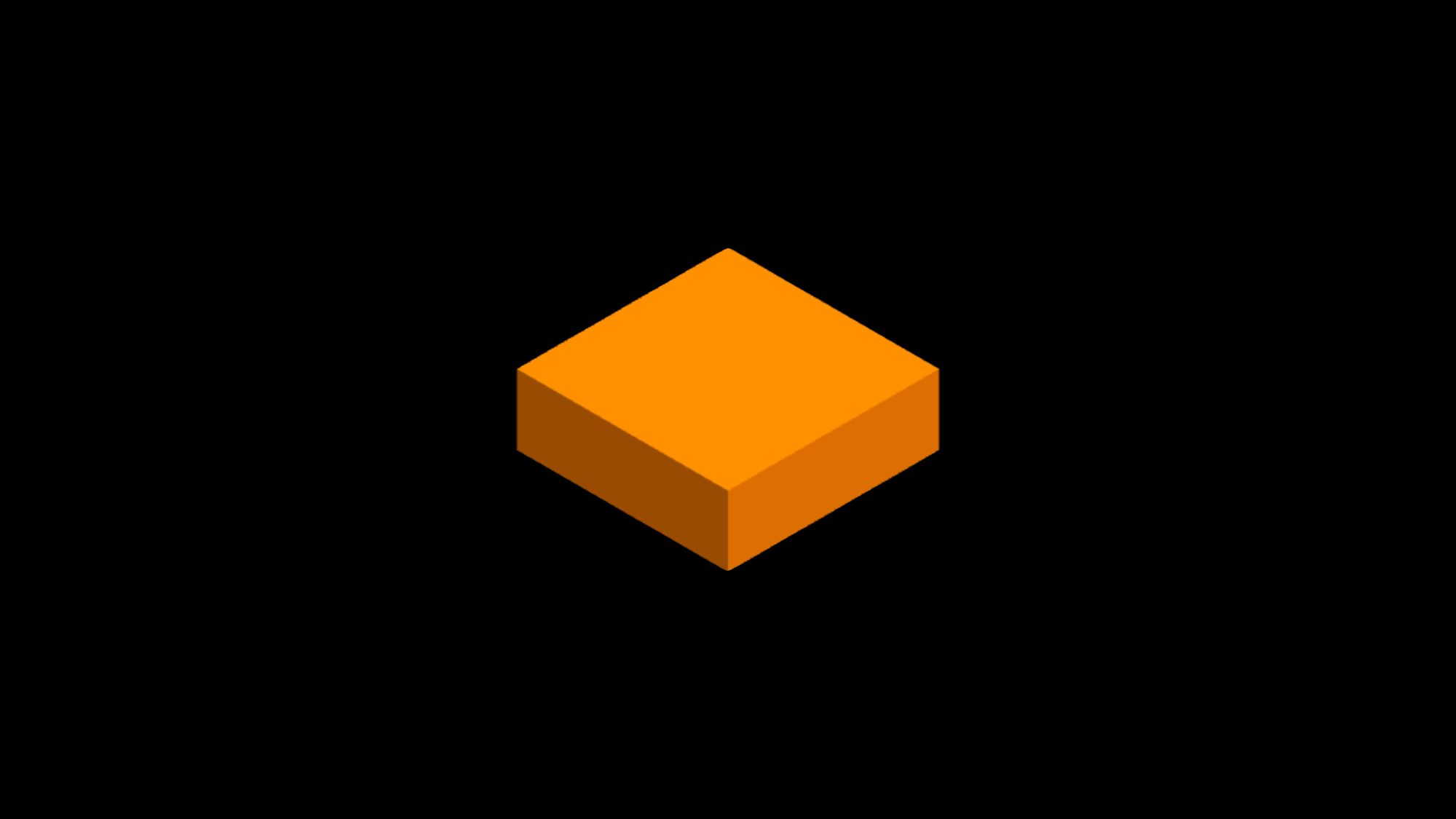
If y'all have ever wanted to build a game with JavaScript, you might accept come across Three.js.
Three.js is a library that we can use to return 3D graphics in the browser. The whole thing is in JavaScript, then with some logic you can add animation, interaction, or even turn information technology into a game.
In this tutorial, we will go through a very simple example. Nosotros'll render a 3D box, and while doing so we'll learn the fundamentals of Three.js.
Three.js uses WebGL under the hood to render 3D graphics. We could use plain WebGL, only it'south very complex and rather low level. On the other hand, Three.js is similar playing with Legos.
In this article, we'll become through how to place a 3D object in a scene, fix the lighting and a camera, and return the scene on a canvas. And so permit's run into how we tin do all this.
Define the Scene Object
Offset, nosotros have to define a scene. This volition be a container where we place our 3D objects and lights. The scene object likewise has some properties, like the background color. Setting that is optional though. If we don't ready it, the default volition be black.
import * as THREE from "iii"; const scene = new THREE.Scene(); scene.groundwork = new Three.Colour(0x000000); // Optional, blackness is default ...
Geometry + Textile = Mesh
Then we add our 3D box to the scene equally a mesh. A mesh is a combination of a geometry and a material.
... // Add a cube to the scene const geometry = new THREE.BoxGeometry(3, i, 3); // width, top, depth const material = new 3.MeshLambertMaterial({ color: 0xfb8e00 }); const mesh = new THREE.Mesh(geometry, material); mesh.position.set up(0, 0, 0); // Optional, 0,0,0 is the default scene.add together(mesh); ...
What is a Geometry?
A geometry is a rendered shape that we're building - like a box. A geometry can exist build from vertices or we can use a predefined one.
The BoxGeometry is the virtually basic predefined selection. We only have to set the width, pinnacle, and depth of the box and that's it.
Y'all might remember that we can't get far by defining boxes, but many games with minimalistic design use only a combination of boxes.
In that location are other predefined geometries likewise. We can easily ascertain a plane, a cylinder, a sphere, or even an icosahedron.
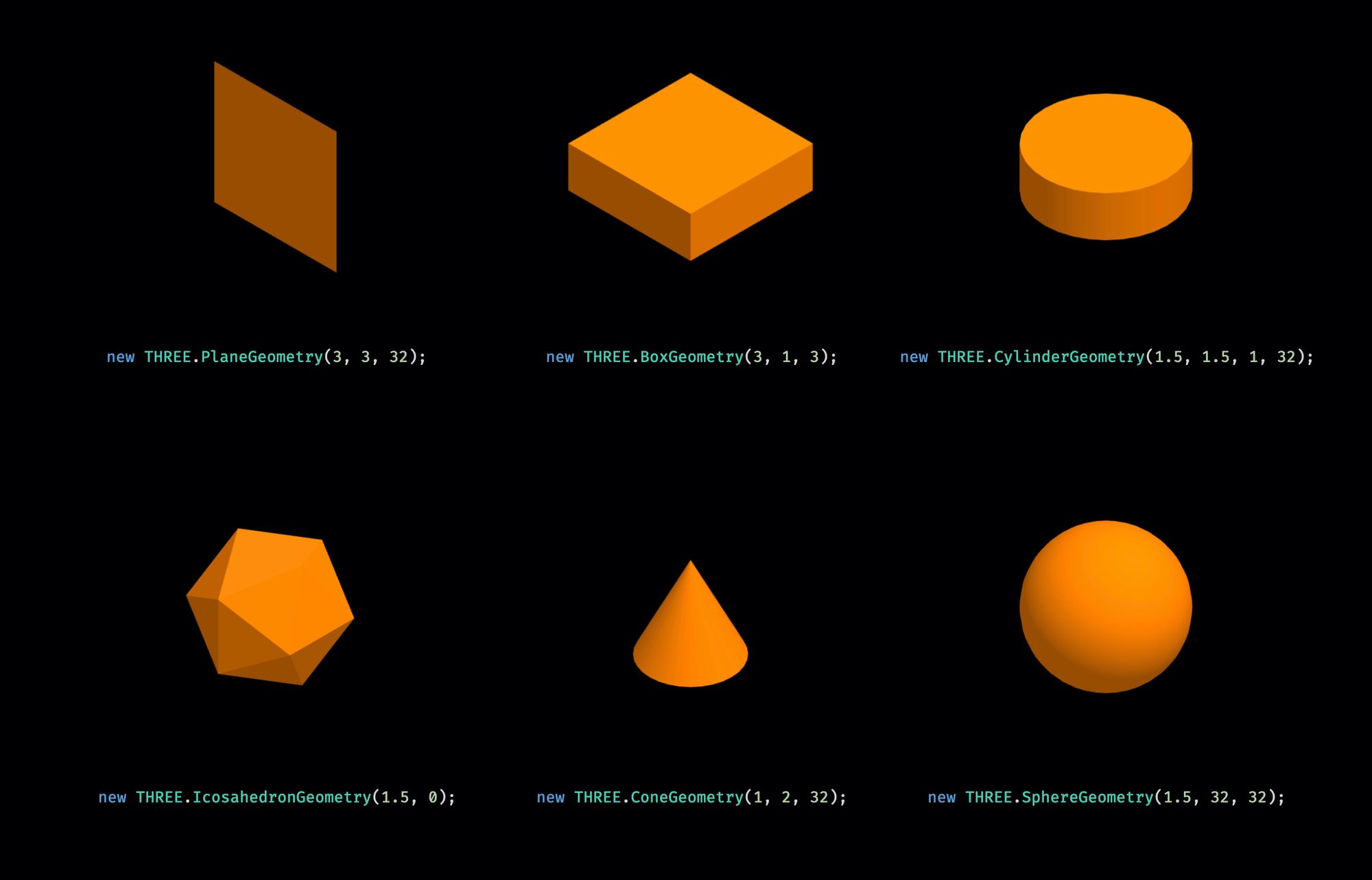
How to Piece of work with Textile
So we ascertain a material. A material describes the appearance of an object. Here we can define things like texture, color, or opacity.
In this instance we are just going to set a color. There are all the same different options for materials. The master difference between virtually of them is how they react to low-cal.
The simplest one is the MeshBasicMaterial. This cloth doesn't care almost lite at all, and each side will accept the same color. It might not be the best option, though, as you can't run across the edges of the box.
The simplest material that cares about light is the MeshLambertMaterial. This will calculate the color of each vertex, which is practically each side. Simply it doesn't go beyond that.
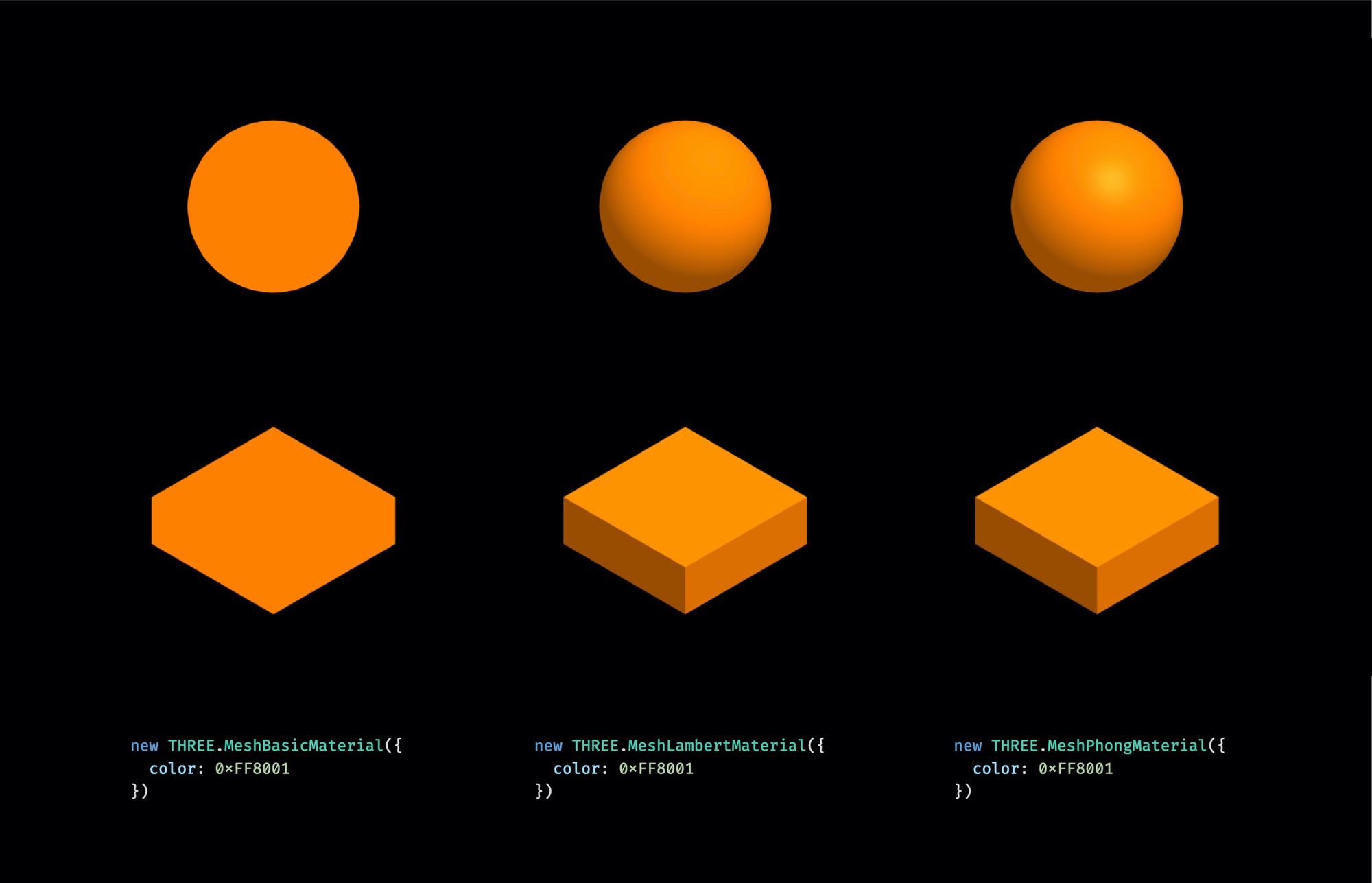
If yous demand more precision, there are more than avant-garde materials. The MeshPhongMaterial not but calculates the color by vertex but past each pixel. The colour tin can change within a side. This tin can help with realism simply also costs in operation.
It also depends on the light settings and the geometry if information technology has whatsoever real upshot. If we render boxes and use directional light, the issue won't change that much. Only if nosotros return a sphere, the difference is more obvious.
How to Position a Mesh
In one case nosotros have a mesh nosotros can also position it inside the scene and ready a rotation by each axis. Later if we desire to animate objects in the 3D infinite we will mostly adjust these values.
For positioning nosotros use the aforementioned units that nosotros used for setting the size. It doesn't affair if y'all are using small numbers or big numbers, you lot but need to be consistent in your ain world.
For the rotation we set the values in radians. So if you have your values in degrees you accept to divide them by 180° and then multiply past PI.

How to Add Calorie-free
Then let'south add together lights. A mesh with basic material doesn't need any low-cal, as the mesh will have the ready color regardless of the light settings.
Simply the Lambert textile and Phong material crave light. If in that location isn't whatsoever light, the mesh will remain in darkness.
... // Fix up lights const ambientLight = new Iii.AmbientLight(0xffffff, 0.6); scene.add(ambientLight); ...
Nosotros'll add together two lights - an ambient light and a directional low-cal.
Kickoff, we add together the ambience light. The ambient light is shining from every direction, giving a base color for our geometry.
To set an ambient calorie-free we set a color and an intensity. The color is usually white, but you tin set any color. The intensity is a number between 0 and i. The two lights we define work in an accumulative way so in this case we want the intensity to be around 0.5 for each.

The directional light has a similar setup, merely information technology also has a position. The word position hither is a bit misleading, because information technology doesn't mean that the light is coming from an verbal position.
The directional low-cal is shining from very far abroad with many parallel light rays all having a fixed bending. But instead of defining angles, we define the management of a single light ray.
In this case, it shines from the management of the 10,twenty,0 position towards the 0,0,0 coordinate. But of class, the directional calorie-free is non only ane light ray, but an infinite amount of parallel rays.
Think of it every bit the sun. On a smaller calibration, light rays of the dominicus as well come downwards in parallel, and the lord's day'southward position isn't what matters but rather its direction.
And that's what the directional light is doing. It shines on everything with parallel calorie-free rays from very far abroad.
... const dirLight = new THREE.DirectionalLight(0xffffff, 0.half-dozen); dirLight.position.gear up(10, xx, 0); // 10, y, z scene.add together(dirLight); ...
Here we gear up the position of the light to be from in a higher place (with the Y value) and shift it a scrap along the X-axis likewise. The Y-centrality has the highest value. This means that the pinnacle of the box receives the most low-cal and it volition be the shiniest side of the box.
The light is also moved a bit along the X-centrality, so the right side of the box will besides receive some light, but less.
And because we don't move the low-cal position forth the Z-axis, the front side of the box will not receive any light from this source. If there wasn't an ambient calorie-free, the forepart side would remain in darkness.
There are other low-cal types as well. The PointLight, for example, can be used to simulate light bulbs. Information technology has a fixed position and it emits light in every management. And the SpotLight can be used to simulate the spotlight of a machine. It emits lite from a single point into a direction along a cone.
How to Set up the Camera
So far, we have created a mesh with geometry and fabric. And we have as well ready up lights and added to the scene. We still need a camera to define how we look at this scene.
At that place are ii options here: perspective cameras and orthographic cameras.
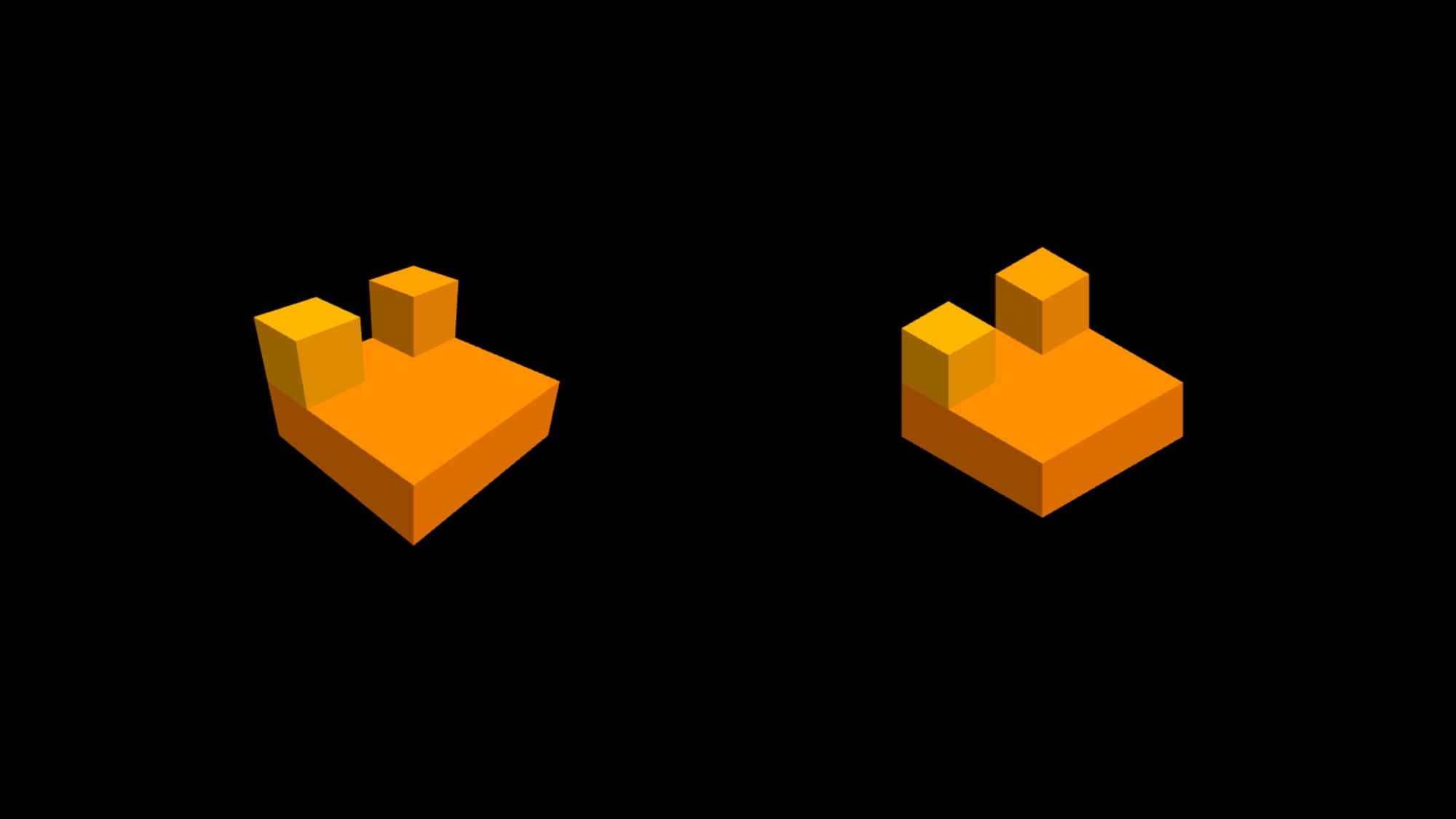
Video games mostly employ perspective cameras, because how they piece of work is similar to how you lot see things in real life. Things that are further abroad announced to exist smaller and things that are right in front of you appear bigger.
With orthographic projections, things will have the same size no affair how far they are from the camera. Orthographic cameras have a more minimal, geometric look. They don't misconstrue the geometries - the parallel lines will appear in parallel.
For both cameras, we have to define a view frustum. This is the region in the 3D space that is going to be projected to the screen. Annihilation exterior of this region won't appear on the screen. This is because it is either too close or besides far away, or because the camera isn't pointed towards it.

With perspective projection, everything within the view frustum is projected towards the viewpoint with a straight line. Things further away from the camera appear smaller on the screen, considering from the viewpoint yous can run into them under a smaller angle.
... // Perspective camera const attribute = window.innerWidth / window.innerHeight; const camera = new Three.PerspectiveCamera( 45, // field of view in degrees aspect, // aspect ratio one, // virtually plane 100 // far aeroplane ); ...
To define a perspective camera, you demand to set up a field of view, which is the vertical angle from the viewpoint. Then yous define an attribute ratio of the width and the height of the frame. If you fill the whole browser window and yous want to keep its aspect ratio, and then this is how yous can exercise information technology.
And then the last two parameters define how far the nearly and far planes are from the viewpoint. Things that are too shut to the camera will exist ignored, and things that are too far away will be ignored as well.
... // Orthographic camera const width = 10; const superlative = width * (window.innerHeight / window.innerWidth); const camera = new 3.OrthographicCamera( width / -2, // left width / 2, // right height / 2, // summit peak / -2, // bottom 1, // nearly 100 // far ); ...
Then there's the orthographic camera. Here we are not projecting things towards a single indicate merely towards a surface. Each projection line is in parallel. That's why it doesn't matter how far objects are from the camera, and that'south why information technology doesn't distort geometries.
For orthographic cameras, we take to define how far each plane is from the viewpoint. The left plane is therefor 5 units to the left, and the correct plane is five units to the correct, and and then on.
... photographic camera.position.set(four, 4, four); camera.lookAt(0, 0, 0); ...
Regardless of which photographic camera are we using, nosotros also demand to position it and set information technology in a direction. If we are using an orthographic photographic camera the bodily numbers here don't affair that much. The objects will appear the same size no matter how far away they are from the camera. What matters, though, is their proportion.
Through this whole tutorial, we saw all the examples through the same camera. This camera was moved by the same unit along every axis and it looks towards the 0,0,0 coordinate. Positioning an orthographic camera is similar positioning a directional lite. It'southward non the actual position that matters, just its direction.
How to Render the Scene
So we managed to put together the scene and a camera. Now only the final piece is missing that renders the image into our browser.
We need to define a WebGLRenderer. This is the piece that is capable of rendering the bodily paradigm into an HTML sail when we provide a scene and a camera. This is likewise where we can set up the actual size of this canvass – the width and elevation of the sheet in pixels as it should announced in the browser.
import * as Iii from "3"; // Scene const scene = new Iii.Scene(); // Add a cube to the scene const geometry = new Three.BoxGeometry(iii, i, three); // width, summit, depth const material = new THREE.MeshLambertMaterial({ color: 0xfb8e00 }); const mesh = new Three.Mesh(geometry, material); mesh.position.set(0, 0, 0); scene.add together(mesh); // Fix lights const ambientLight = new 3.AmbientLight(0xffffff, 0.6); scene.add together(ambientLight); const directionalLight = new Three.DirectionalLight(0xffffff, 0.6); directionalLight.position.set up(ten, xx, 0); // x, y, z scene.add(directionalLight); // Camera const width = x; const height = width * (window.innerHeight / window.innerWidth); const camera = new THREE.OrthographicCamera( width / -2, // left width / 2, // right pinnacle / ii, // meridian height / -two, // lesser ane, // near 100 // far ); photographic camera.position.set(iv, four, 4); camera.lookAt(0, 0, 0); // Renderer const renderer = new 3.WebGLRenderer({ antialias: true }); renderer.setSize(window.innerWidth, window.innerHeight); renderer.return(scene, camera); // Add information technology to HTML document.torso.appendChild(renderer.domElement);
And finally, the last line here adds this rendered canvas to our HTML document. And that's all you need to render a box. It might seem a little too much for just a single box, just most of these things we simply accept to set up once.
If you want to move forwards with this project, then check out my YouTube video on how to plow this into a elementary game. In the video, we create a stack building game. We add together game logic, event handlers and blitheness, and fifty-fifty some physics with Cannon.js.
If you have whatsoever feedback or questions on this tutorial, feel free to Tweet me @HunorBorbely or leave a comment on YouTube.
Learn to code for free. freeCodeCamp'southward open source curriculum has helped more than twoscore,000 people go jobs every bit developers. Go started
Source: https://www.freecodecamp.org/news/render-3d-objects-in-browser-drawing-a-box-with-threejs/
0 Response to "how to draw open front a 3d box"
Post a Comment